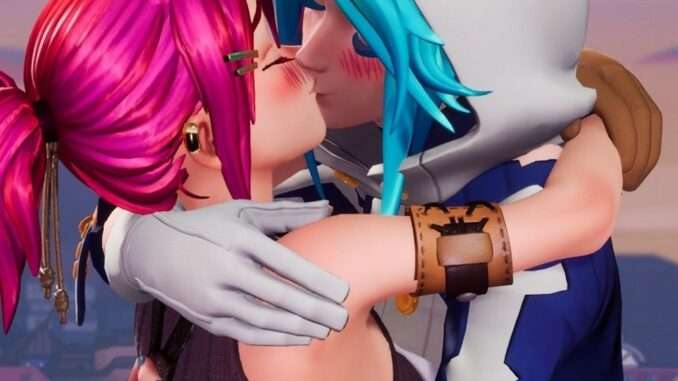
Algorithm
Сrеdit gоеs to NatsuFTryuu !
I like math and optimization problems (which is a pretty weird thing to like I admit), so I decided to apply my skills to this game. I created an algorithm in Mathematica that allows you to find the ingredients that are perfectly balanced.
There is a list of ingredients (along with their magimin and trait values), a list of potions (along with their ratios of the various magimins). You need to specify the amount of ingredients your cauldron holds as well as its maximum magimin value and which potion you want to create.
Having done that, with a simple “Control+A” and “Shift+Enter” it will provide you with the total value of the created potion as well as the ingredients and their number in order to create a perfectly balanced potion with your available ingredients and cauldron constraints. If it produces some weird numbers or no numbers at all, then you don’t have the ingredients to create a perfectly balanced potion.
(*This is meant to run in Mathematica. For those who have it you presumably know how to use it. For those who don't, simply copy-paste this entire thing into it and change what you need to change. (The appropriate spots have been specified). Then simply select everything (Ctrl+A) and execute (Shift+Enter). For anybody who's interested, I hope this is helpful to you.*)
Clear["Global`*"]
(*Ingredients array*)
(*You can add more ingredients yourself using the same format*)
ingredients = {
{"feyberry", {6, 0, 0, 0, 0}, {0, 0, 0, 0, 0}},
{"mandrake_root", {0, 6, 0, 0, 0}, {0, 0, 0, 0, 0}},
{"sack_of_slime", {0, 0, 6, 0, 0}, {0, 0, 0, 0, 0}},
{"pixiedust_diamond", {0, 4, 4, 0, 0}, {0, 0, 0, 0, 0}},
{"unicorn_horn", {0, 0, 8, 0, 0}, {-1, 0, 0, 0, 0}},
{"rotfly_larva", {0, 0, 4, 0, 0}, {1, 0, 0, 0, 0}},
{"river-pixie's_shell", {4, 4, 0, 0, 0}, {0, 0, 0, 0, 0}},
{"leech_snail's_shell", {12, 12, 0, 0, 0}, {0, 0, 0, 0, 0}},
{"horned_jelly", {18, 0, 0, 0, 0}, {0, -1, 0, 1, 0}},
{"sphinx_flea", {12, 6, 0, 0, 0}, {0, 1, 0, 0, 0}},
{"kappa_pheromones", {4, 0, 4, 0, 0}, {0, 0, 0, 0, 0}},
{"cubic_ooze", {3, 3, 3, 0, 0}, {0, 0, 0, 0, 0}},
{"fairy_flower_bulb", {4, 0, 0, 0, 0}, {0, 0, 1, 0, 0}},
{"river_calamari", {8, 0, 0, 0, 0}, {0, -1, 0, 0, 0}},
{"glass_ore", {0, 0, 0, 18, 0}, {0, 0, 0, 0, 0}},
{"serpent's_slippery_tongue", {0, 8, 0, 0, 0}, {0, 0, -1, 0, 0}},
{"rotfly_cocoon", {0, 0, 12, 0, 0}, {1, 0, 0, 0, 0}},
{"sack_of_hive_slime", {0, 0, 18, 0, 0}, {0, 0, 0, 0, 0}},
{"bog_beet", {0, 27, 0, 0, 0}, {-1, 0, 0, 0, 1}},
{"qilin's_tri_horn", {0, 0, 24, 0, 0}, {-1, 0, 0, 0, 0}},
{"bubble_ooze", {9, 9, 12, 12, 0}, {0, 0, 0, 0, 0}},
{"golem's_eye_diamond", {0, 12, 12, 0, 0}, {0, 0, 0, 0, 0}},
{"manwyrm_root", {0, 18, 0, 0, 0}, {0, 0, 0, 0, 0}},
{"figment_pomme", {0, 18, 6, 0, 0}, {0, 0, 0, 0, 0}},
{"golemite", {18, 12, 0, 10, 0}, {0, 0, 0, 0, 0}},
{"puckberry", {18, 0, 0, 0, 0}, {0, 0, 0, 0, 0}},
{"warg_pheromones", {12, 0, 12, 0, 0}, {0, 0, 0, 0, 0}},
{"murkwater_pearl", {0, 0, 0, 12, 0}, {0, 0, 0, 1, 0}},
{"swamp_fish", {12, 0, 0, 6, 0}, {0, 0, 0, 0, 0}},
{"fairy_flower_bud", {12, 0, 0, 0, 0}, {0, 0, 1, 0, 0}},
{"impstool_mushroom", {0, 4, 0, 0, 0}, {0, 1, 0, 0, 0}},
{"mud_shrimp", {6, 0, 12, 0, 0}, {0, 0, 1, 0, 0}},
{"swamp_octopus", {24, 0, 0, 0, 0}, {0, -1, 0, 0, 0}},
{"desert_metal", {0, 12, 0, 0, 0}, {0, 1, 0, 0, 0}},
{"antlered_jelly", {30, 0, 0, 0, 0}, {0, -1, 0, 1, 0}},
{"static_spiderling", {0, 0, 0, 0, 30}, {0, 0, 0, -1, 1}},
{"cobweb_crayfish", {10, 0, 20, 0, 0}, {0, 0, 1, 0, 0}},
{"hallucinatory_shroom", {0, 0, 30, 0, 0}, {1, 0, 0, 0, -1}},
{"phantom_pomme", {0, 10, 30, 0, 0}, {1, 0, 0, 0, -1}}
};
(*Cauldron constraints*)
(*These can be changed as needed*)
cauldronSize = 8;
cauldronMaxValue = 240;
(*Potion requirements*)
(*More can be added on. It's simply the names and ratios of each magimin*)
potions = {
{"health_potion", {1, 1, 0, 0, 0}},
{"mana_potion", {0, 1, 1, 0, 0}},
{"stamina_potion", {1, 0, 0, 0, 1}},
{"speed_potion", {0, 0,1, 1, 0}},
{"fire_tonic", {1, 0, 1, 0, 0}},
{"ice_tonic", {1, 0,0, 1, 0}},
{"thunder_tonic", {0, 1, 0, 1, 0}},
{"shadow_tonic", {0, 1, 0, 0, 1}},
{"sight_enhancer", {3, 4, 3, 0, 0}},
{"alertness_enhancer", {0, 3, 4, 3, 0}},
{"poison_cure", {2, 0, 1, 1, 0}},
{"drowsiness_cure", {1, 1, 0, 2, 0}}
};
(*Chosen Potion*)
(*Here we put the potion we want to create*)
chosenPotion = {"ice_tonic", {1, 0, 0, 1, 0}};
(*FROM THIS POINT ON, YOU DON'T NEED TO CHANGE ANYTHING.*)
(*Ingredients for chosen potion*)
truthfulness = ConstantArray[False, {Length[ingredients], 5}];
For]], i++,
For[k = 1, k <= Length[ingredients], k++,
If[chosenPotion[[2]][[i]] != 0,
If[ingredients[[k, 2]][[i]] >= 0,
truthfulness[[k, i]] = True,
truthfulness[[k, i]] = False
],
If[ingredients[[k, 2]][[i]] != 0,
truthfulness[[k, i]] = False,
truthfulness[[k, i]] = True
]
];
];
];
acceptableIngredients = Array[0, {Length[ingredients], 3}];
For[j = 1, j <= Length[ingredients], j++,
If[truthfulness[[j]] == {True, True, True, True, True},
acceptableIngredients[[j]] = {ingredients[[j, 1]],
ingredients[[j, 2]], ingredients[[j, 3]]},
acceptableIngredients[[j]] = {0, 0, 0}
];
];
sum = ConstantArray[0, 5];
variables = Array[x, Length[acceptableIngredients]];
For[j = 1, j <= 5, j++,
For, i++,
If[Total[acceptableIngredients[[i, 2]]] != 0,
sum[[j]] += acceptableIngredients[[i, 2]][[j]]*variables[[i]]
];
];
];
(*Formulate the optimization problem*)
constraints = {
Total[Total[acceptableIngredients[[All, 2]]*variables]] <=
cauldronMaxValue,
Total[variables] <= cauldronSize,
sum[[1]] <= (chosenPotion[[2]][[1]]/Total[chosenPotion[[2]]])*
Total[Total[acceptableIngredients[[All, 2]]*variables]],
sum[[2]] <= (chosenPotion[[2]][[2]]/Total[chosenPotion[[2]]])*
Total[Total[acceptableIngredients[[All, 2]]*variables]],
sum[[3]] <= (chosenPotion[[2]][[3]]/Total[chosenPotion[[2]]])*
Total[Total[acceptableIngredients[[All, 2]]*variables]],
sum[[4]] <= (chosenPotion[[2]][[4]]/Total[chosenPotion[[2]]])*
Total[Total[acceptableIngredients[[All, 2]]*variables]],
sum[[5]] <= (chosenPotion[[2]][[5]]/Total[chosenPotion[[2]]])*
Total[Total[acceptableIngredients[[All, 2]]*variables]],
variables \[Element] PositiveIntegers
};
(*Solve the optimization problem*)
solution =
NMaximize[{Total[Total[acceptableIngredients[[All, 2]]*variables]],
constraints}, variables];
(*Display the result*)
ingredientQuantities = variables /. solution[[2]];
(*Extract the names of the ingredients needed for the potion*)
neededIngredients =
Select[Transpose[{ingredients[[All, 1]],
ingredientQuantities}], #[[2]] > 0 &];
Print["The ingredients needed to make the '", chosenPotion[[1]], "' potion with a total value of ", solution[[1]]];
Print[neededIngredients];[/code]
Be the first to comment